HTML DOM Simplified
JavaScript HTML DOM Simplified, Beginnerβs Guide
The Document Object Model (DOM) is a tree-like structure containing all the elements and content of a web page. It enables you to manipulate the content of a webpage in real-time using JavaScript. With DOM, you can create interactive and dynamic user interfaces.
In this article, youβll learn how to create event listeners and select, create, modify, and delete elements and attributes on a web page.
Accessing DOM elements in JavaScript
β
The HTML DOM enables you to access every element on a web page with JavaScript, and there are five ways by which you can access these elements. Before going over them, create a simple web page as shown below.
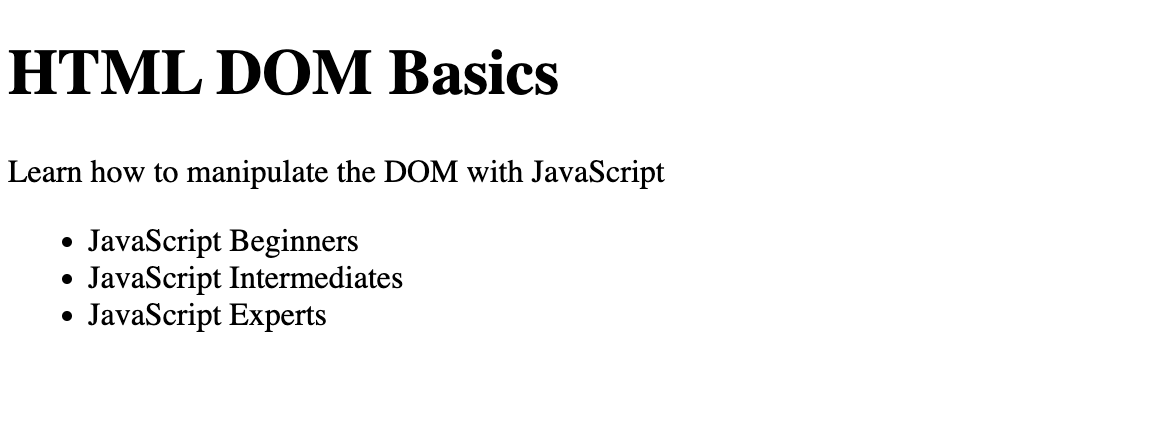
<h1 id="heading">HTML DOM Basics</h1>
<p id="paragraph">Learn how to manipulate the DOM with JavaScript</p>
<ul class="list">
<li class="item">JavaScript Beginners</li>
<li class="item">JavaScript Intermediates</li>
<li class="item">JavaScript Experts</li>
</ul>
π Using getElementById()
β
This method allows you to access
an HTML element via its ID Attribute
. It returns an entire element or null, if the element does not exist.
The code snippet below selects the HTML element with an id of heading from the web page.
const heading = document.getElementById("heading");
console.log(heading); // ππ» Output: <h1 id="heading">HTML DOM Basics</h1>
π Using getElementsByClassName()
β
With document.getElementsByClassName()
, you can access elements using their Class Attribute
.
It returns an array-like object called an HTMLCollection
that contains all the elements with the specified class name.
const item = document.getElementsByClassName("item");
console.log(item); // ππ» Output: HTMLCollection(3)
π Using getElementsByTagName()
β
This method allows you to access
HTML elements via their Tag Name
. It returns an HTMLCollection
containing all the elements with the same tag name.
const paragraph = document.getElementsByTagName("p");
console.log(paragraph); // ππ» Output: HTMLCollection(1)
The paragraph
variable contains an array of all the paragraph tags on the web page.
π Using querySelector()
β
With this method, you can access an element via its CSS Selector
. It returns the first element that matches the selector.
const paragraph = document.querySelector("#paragraph");
console.log(subHeading); // ππ» Output: <p id="paragraph">Learn how to manipulate the DOM with JavaScript</p>
const list = document.querySelector(".list");
console.log(list); // ππ» Output: <ul class="list">...</ul>
The above code snippet shows you can reference an element via its ID
or class attributes
.
Using querySelectorAll()
β
It is similar to the document.querySelector
method but allows you to access multiple elements using a CSS selector.
It returns a NodeList
of all the HTML elements that match the selector.
const items = document.querySelectorAll(".item");
console.log(items); // ππ» Output: NodeList(3)
The code snippet above returns all the HTML elements with a class attribute of item
.
Modifying
DOM Elementsβ
One of the most powerful features of JavaScript is its ability to alter HTML elements on a web page in real-time without reloading the entire page.
Here, you'll learn how to modify HTML elements with JavaScript
.
π Using the textContent
propertyβ
The textContent
property allows you to change only the content of an HTML element.
const header = document.querySelector("#heading");
header.textContent = "HTML DOM Manipulation";
The code snippet above changes the content of the h1
tag to "HTML DOM Manipulation".
π Using the innerHTML
propertyβ
It allows you to modify the content, tags, and attributes of an HTML element. You change the p
tag to a h3
tag, as shown below.
const paragraph = document.getElementById("paragraph");
paragraph.innerHTML = "<h3>DOM Manipulation made easy</h3>";
Adding Elements
to the HTML DOMβ
To add new elements to the HTML DOM via JavaScript, you can use the createElement()
method and the appendChild()
method.
The createElement()
method creates a new element with JavaScript, and the appendChild()
adds the newly created element to a parent element on the HTML DOM.
You can add a new list item to the ul
tag on the web page as done below.
const list = document.querySelector(".list");
const item = document.createElement("li");
item.textContent = "Newly added";
item.className = "item";
list.appendChild(item);
console.log(item); // ππ» Output: <li class="item">Newly added</li>
The code snippet above,
- gets the
ul
element from the web page, - creates a new
li
element, sets its content, and adds a class attribute to theli
element, then, - appends the
li
element to theul
tag on the web page.
Removing Elements
from the HTML DOMβ
To remove elements from the HTML DOM in JavaScript, you can use the removeChild()
method.
This method allows you to remove a child element from its parent element.
const list = document.querySelector(".list");
const firstItem = document.querySelector(".item");
console.log(firstItem); //Output: ππ» <li class="item">JavaScript Beginners</li>
list.removeChild(firstItem);
The code snippet above gets the entire unordered list and its first child from the web page and removes the first child from the list using the removeChild()
method.
Listening
to DOM events with JavaScriptβ
The HTML DOM also allows you to create event listeners that listen to actions performed on a particular element on the web page. You can listen to various events carried out by users on a webpage.
Add a button element to the web page. We'll add event listeners to the button.
<h1 id="heading">HTML DOM Basics</h1>
<p id="paragraph">Learn how to manipulate the DOM with JavaScript</p>
<ul class="list">
<li class="item">JavaScript Beginners</li>
<li class="item">JavaScript Intermediates</li>
<li class="item">JavaScript Experts</li>
</ul>
<button id="button">Hello Reader</button>
π‘ Note: Every element has its set of events. You can add event listeners to several HTML elements, including buttons, links, input fields, and more.
π Using the addEventListener()
methodβ
The addEventListener()
method is a built-in method in JavaScript that allows you to add event listeners to HTML elements. It takes two arguments: the type of event to listen for (such as click, mouseover, keydown, etc.) and the function to execute when the event occurs.
const button = document.getElementById("button");
button.addEventListener("click", function () {
alert("Button Clicked!");
});
The code snippet above references the button element and listens for a click event on the button. Once a user clicks the button, it triggers the alert
method.
π Using the element.event
methodβ
It is an older way of adding event listeners to HTML elements in JavaScript. It assigns a function to an HTML element.
const button = document.getElementById("button");
button.onclick = function () {
alert("Button Clicked!");
};
The button.onclick
method may look straightforward compared to the addEventListener
method, but it has some limitations.
π addEventListener()
vs element.event
β
- The
addEventListener
method can execute multiple functions when a particular event occurs; this is beneficial when building complex or interactive web applications. Inelement.event
, only a single function can be attached to an event.
const button = document.getElementById("button");
button.addEventListener("click", function () {
console.log("Button Clicked!");
});
button.addEventListener("click", function () {
console.log("JavaScript is easy!");
});
//ππ» Both functions are executed
From the code snippet above, both functions are executed when a user clicks the button. Below is an example using the element.event
method; the last event overrides the previous one.
const button = document.getElementById("button");
button.onclick = function () {
console.log("First click!");
};
button.onclick = function () {
console.log("JavaScript is easy");
};
//ππ» only the last event is executed
The addEventListener()
method provides more control over the execution of the event handlers.
You can remove an event using the removeEventListener
method.
β In Summaryβ
So far, youβve learnt the basics of what the DOM is and how it works, how to modify elements, add new ones, and listen to events on your web page. A solid understanding of the HTML DOM is essential when building interactive web pages and creating dynamic user interfaces with HTML and JavaScript.
β Resourcesβ
- π Access AppSeed for more starters and support
- π Deploy Projects on Aws, Azure and DO via DeployPRO
- π Create landing pages with Simpllo, an open-source site builder
- π Build apps with Django App Generator (free service)